In 2017, we checked out devkitSMS Programming Setup to build 8-bit game code for the Master System. As we upgrade to the 16-bit MD we would like to replicate this process now using Sega Genesis Dev Kit [SGDK].

Let’s check it out!
SGDK
The SGDK is a free development kit allowing you to develop software in C language for the Sega Mega Drive. Note that SGDK also requires Java (custom tools need it) so you need to have Java installed on your system.
IMPORTANT
Ensure an up-to-date version of Java is installed, e.g. version "12.0.2" 2019-07-16 otherwise builds may fail!
Software
Follow most instructions from the previous post: this documents how to setup some pre-requisite software.
Here is a summary of some of the software to be installed:
|
|
Gens KMod "gens" can also be built from source. Add gens.exe to Gens folder similar to Fusion in this post. Note: you may already have gcc configured on you computer if you previously installed cygwin or Git Bash

SGDK
Navigate to the SGDK repository on github: @MegadriveDev has full instructions here. Download the latest release from SGDK archive e.g. 1.51 or simply git clone the latest source code. Extract code into C:\SGDK.
Setup the following environment variables for GDK + GDK_WIN to be used throughout SGDK development. Add the following two environment variables: System | Advanced system settings | Environment Variables:
Variable | Value | Description |
GDK | C:/SGDK | UNIX path format |
GDK_WIN | C:\SGDK | Windows path format |
Next, Stephane advises to add the %GDK_WIN%\bin to your PATH variable being careful if you have another GCC installation as you may have conflicts. I have multiple GCC installs but have not experienced any issues.
Finally, generate the SGDK library %GDK%/lib/libmd.a by entering the following command Start | run | cmd.
%GDK_WIN%\bin\make -f %GDK_WIN%\makelib.gen
Example
As an example, let's write a simple program that prints "Hello World" using SGDK. Create new directory: C:\HelloWorld. Create main.c file + enter the following code similar to the Hello World tutorial program.
main.c
#include <genesis.h> int main() { VDP_drawText( "Hello Genny World!", 10, 13 ); while( 1 ) { VDP_waitVSync(); } return 0; }
Build
Manually compile, link and execute the Hello World program. Launch command prompt: Start | Run | cmd.

Change directory cd C:\HelloWorld. Next, execute the following make command to produce the output ROM:
ACTION | COMMAND | OUTPUT |
Compile | %GDK_WIN%\bin\make -f %GDK_WIN%\makefile.gen | out\rom.bin |
Finally, type out\rom.bin. The Hello World program should launch in the Fusion emulator.
Congratulations! You have just written your first Mega Drive program using the SGDK.
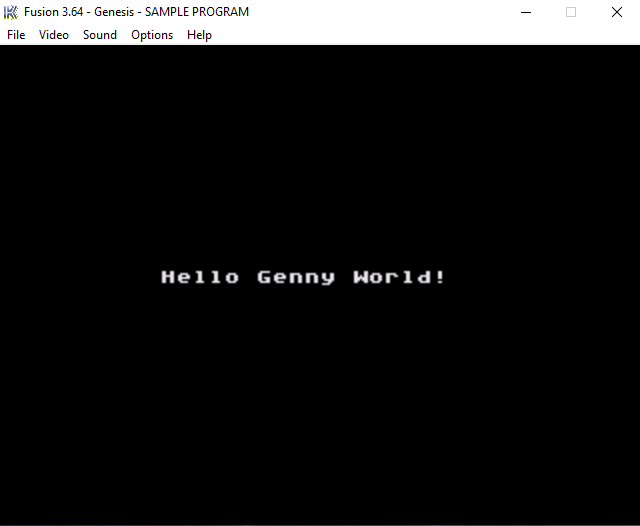
Automate
Let's automate the build process: create C:\HelloWorld\build.bat script file that contains the commands:
@echo off %GDK_WIN%\bin\make -f %GDK_WIN%\makefile.gen out\rom.bin
Code Blocks
Follow all instructions here to setup SGDK with CodeBlocks. @matteusbeus has great video on how to setup SGDK using CodeBlocks also. Check out his YouTube channel for other interesting videos with SGDK topics.
Eclipse
Follow all instructions here to setup SGDK with Eclipse. @SpritesMind has a great tutorial on how to remote debug SGDK code using Gens KMod with Eclipse also. Follow all instructions here to complete debug setup:
Launch Eclipse. Choose Run menu | Debug Configurations | C/C++ Remote Application | New Configuration

Name | Remote Debugging |
Project | HelloWorld |
C/C++ Application | C:\HelloWorld\out\rom.out |
Disable auto build | CHECKED |

Stop on startup as: main | CHECKED |
GDB debugger | C:SGDK\bin\gdb.exe |
GDB command line | .gdbinit |

Type | TCP |
Host name or IP address | localhost |
Port number | 6868 |
Visual Studio Code
@ohsat_games has a great tutorial on how to setup SGDK using Visual Studio Code. You can even install the Genesis Code extension for code auto completion and to streamline the build process all from within the IDE.
Further SGDK tutorials from @ohsat_games can be found here. All projects have a similar build batch script:
%GDK_WIN%\bin\make -f %GDK_WIN%\makefile.gen
Visual Studio 2015
Retro Mike has a great video on how to setup SGDK using Visual Studio 2015. Launch Visual Studio 2015. File | New | Project... | Visual C++ | General | Makefile Project. Enter the following name + location info:

Name: | HelloWorld |
Location: | C:\ |
Create directory for solution | UNCHECKED |
Choose Next. Enter the following Build command: %GDK_WIN%\bin\make -f %GDK_WIN%\makefile.gen

Choose Finish. Enter the previous "Hello World" code as above. Finally, right click Project | Properties | VC++ Directories | Include Directories. Enter the following in order to include SGDK header files: $(GDK_WIN)\inc

Press Ctrl + Shift + B to build source code and produce out\rom.bin. Launch Fusion emu + load ROM file! Alternatively, setup "Remote" debugging configuration in Visual Studio and Press Ctrl + F5 to build and run.

Right click Project | Properties | Debugging | Remote Windows Debugger. Update in the following properties:
Remote Command | C:\SEGA\Fusion\Fusion.exe |
Remote Command Arguments | out\rom.bin |
Connection | Remote with no authentication |
Debugger Type | Native Only |
Attach | Yes |
Summary
To summarize, there are multiple IDE setups available to use the SGDK. However, despite VS2015 being the most applicable to our previous devkitSMS process, we're still not able to debug step thru the C source code.
Ultimately, we would like to build + debug step thru the C source code during SGDK development whilst also being able to build + run the ROM output file all from in Visual Studio. This will be the topic of the next post.